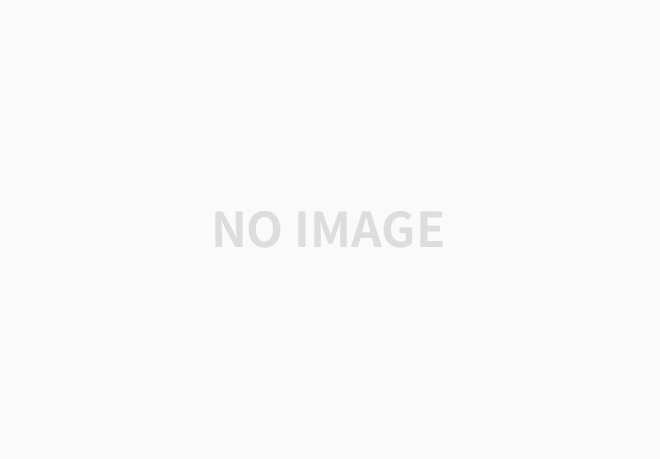
name='aaa'
email='bbb@gmail.com'
addr='seoul'
def print_business_card(name,email,addr):
print('name:%s'%name)
print('email:%s'%email)
print('address:%s'%addr)
print_business_card(name,email,addr)
>>>
name:aaa
email:bbb@gmail.com
address:seoul
- parameter
A parameter is the variable listed inside the parentheses in the function definition.
- argument
An argument is the value that is sent to the function when it is called.
def add(a, b): # a, b are parameters
return a+b
print(add(3, 4)) #3, 4 are arguments
- function(depend on input, output)
input(fruits)-->function(blender)-->output(juice)
Basically, there are 4 types depend on whether there is input, output or not.
1. basic function
Thre are input, output.
def add(a, b): #add is function
result = a + b
return result
a = add(3, 4) #variable=function(argument1, argument2), argument1, argument2 are input
print(a)
7 # output
2. function without input
def say(): #there is no input within ()
print('Hi')
a = say()
>>> print(a) #output
Hi
3. function without output
def add(a,b): #here is input
print("%d add %d is %d"%(a,b,a+b)) #there is no return, in other words, no output
#output is only returned return
>>>add(3,4)
3 add 4 is 7
4. function which has neither input or output
def say(): #there is no input within ()
print('hi') #no output niether
>>>say()
hi
- function(unsure input)
1. *args
Arguments, It returns if there is index type(number) as tuple. It must be placed after variable.
def abc(*args):
return args[1],args[0],args[2]
abc(*(1,3,5))
>>>
(3, 1, 5)
def add_many(*args): #function add_many doesn't matter how many input if there is * in front of parameter
# * return tuple type
result=0
for i in args:
result=result+i
return result
result=add_many(1,2,3)
>>>result
6
def abc(a,*args):
return args[0],a,args[1]
abc(*(5,7,9))
>>>
(7, 5, 9)
2. **kwargs
KeyWorded Argument, it returns key, value.
def air_line(depart, arrival, flight):
print(depart)
print(arrival)
print(flight)
myflight={'depart':'seoul','arrival':'aaa','flight':'10'}
air_line(**myflight)
>>>
seoul
aaa
10
air_line(**{'depart':'seoul','arrival':'aaa','flight':'10'})
>>>
seoul
aaa
10
def air_line(**kwargs):
print(kwargs['depart'])
print(kwargs['arrival'])
print(kwargs['flight'])
air_line(**{'depart':'seoul','arrival':'aaa','flight':'10'})
>>>
seoul
aaa
10
def air_line(depart,**kwargs):
print(depart)
print(kwargs['arrival'])
print(kwargs['flight'])
air_line(**{'depart':'seoul','arrival':'aaa','flight':'10'})
>>>
seoul
aaa
10
- variable, *variable, **variable (Always in order like this.)
def blog_printer(name, *blogs, **blog_benefits):
print(name)
for post in blogs:
print(post)
for blog, benefits in blog_benefits.items():
print(blog,'is', benefits)
name='aaa'
b1='first'
b2='second'
b3='third'
blog_printer(name,b1,b2,b3, benefit1=10,benefit2=20,benefit3=30)
>>>
aaa
first
second
third
benefit1 is 10
benefit2 is 20
benefit3 is 30
3. *value
It returns any values.
def test(*val):
for i in val:
print(i)
>>>test(1,'d',4,'f',1.5,2*5)
1
d
4
f
1.5
10
- usage of return
1. It returns tuple type if there are more than one arguments.
def add_and_mul(a,b):
return a+b, a*b
>>>add_and_mul(3,4)
(7, 12)
2. It escapes immediate when it encounters return.
def say_nick(nick):
if nick=='aaa':
return
print('%s'%nick)
>>>say_nick('aaa')
#there is no return, because say_nick function escape when encounter 'aaa'
3. It returns any object as return value.
def calc(n1,n2,func):
if n1>0 and n2>0:
return func(n1,n2)
else:
return 'negative'
def mul(v1,v2):
return v1*v2
>>>calc(3,4,mul) #it returns another def
12
- Initialize parameter
def say(name,old,man=True): #initialize man=True in parameter. It used in the case that parameter doesn't change
print('name is %s' %name)
print('age is %d' %old)
if man:
print('man')
else:
print('ddd')
>>>say('asdf',34)
name is asdf
age is 34
man
>>>say('daf',12,False)
name is daf
age is 12
ddd
def add_book(book_list=None):
if book_list is None:
book_list=[]
book_list.append('python')
return book_list
book_list=['aaa','vvv']
add_book(book_list)
add_book()
>>>
['python']
>>>
['python']
- Usage of global variable
1. Usage return
a=1 #this is variable outside of function
def var(a):
a=a+3 #there is no return
var(a)
>>>a
1 #this returns variable a
a=1
def test(a):
a=a+3
return a
a=test(a) #a changes result of function a
>>>a
4 #therefore it returns 4
#but there are still different betwwen variable a outside of funtion test and variable a inside of function test
2. Usage global(not recommendable)
a=1
def test():
global a #When it uses global, it going to use outside variable a
a=a+4
test()
>>>a
5
x=1
def a():
x=10
def b():
x=20
def c():
global x #whatever variable is here, always refer global x variable
x=x+30
print(x)
c()
b()
>>>a()
31
3. Modifiable from local to global
If there is no global variable but local, it can be local when local defined to global within function.
#here is no global variable
def fo():
global x #define global variable within function, then it can be the global variable
x=20
print(x)
>>>fo()
>>>print(x)
20
20
- Usage of nonlocal
def a():
x=10
y=100
def b():
x=20
def c():
nonlocal x #using local variable x in b()
nonlocal y #using local variable y in a()
x=x+30
y=y+300
print(x)
print(y)
c()
b()
>>>a()
50
400
- namespace
def fo():
x=10
print(locals())
>>>fo()
{'x': 10}
'Basic Python' 카테고리의 다른 글
copy, copy.copy, copy.deepcopy, get, next, id (0) | 2021.05.04 |
---|---|
Module, __name__, __main__ (0) | 2021.04.27 |
Class, pass, instance, binding, Object, variable, self, method, __init__, dir, namespace, __dict__, __del__ (0) | 2021.04.20 |
with as, open, dump, load, contextlib (0) | 2020.12.26 |
try, except, else, finally, sys.stderr, raise (0) | 2020.12.16 |