How can load external data and represents them as a bar chart?
- JSON file
File name is mydata.json
[
{"name":"maria", "age":30},
{"name":"fred", "age":50},
{"name":"francis", "age":10}
]
- HTML document
<!DOCTYPE html>
<html lang="en">
<head>
<title>D3js</title>
<script src="https://d3js.org/d3.v6.min.js"></script>
</head>
<body>
<script>
d3.json("mydata.json").then(function(data){ //function is called a callback function meaning all the code within {} that depends on the data being available should be stored within this function
//data in turn references the data stored in my file which specify in mydata.json
var canvas = d3.select("body").append("svg")
.attr("width", 500)
.attr("height", 500)
canvas.selectAll("rect")
.data(data) //it referencing the data variable that I define up as an argument to callback function
.enter()
.append("rect")
.attr("width", function (d) {return d.age*10;})
.attr("height", 48) //reduce the height with two px
.attr("y", function (d,i){return i*50;})
.attr("fill","orange")
canvas.selectAll("text")
.data(data)
.enter()
.append("text")
.attr("fill","white")
.attr("y", function (d,i){return i*50+24;})
.text(function (d){return d.name;})
})
</script>
</body>
</html>
- Result
Each bar represents the age of each person from mydata.json.
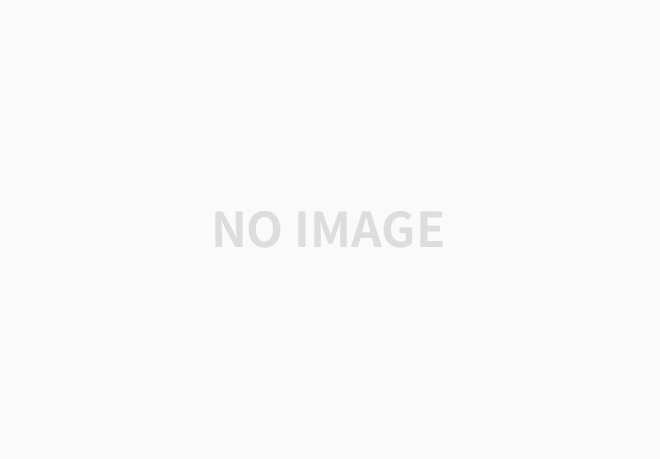
'd3.js' 카테고리의 다른 글
arc (0) | 2020.12.29 |
---|---|
path (0) | 2020.12.29 |
interaction between html document and console (0) | 2020.12.28 |
duration, delay, transition, on (0) | 2020.12.28 |
enter, update, exit (0) | 2020.12.28 |